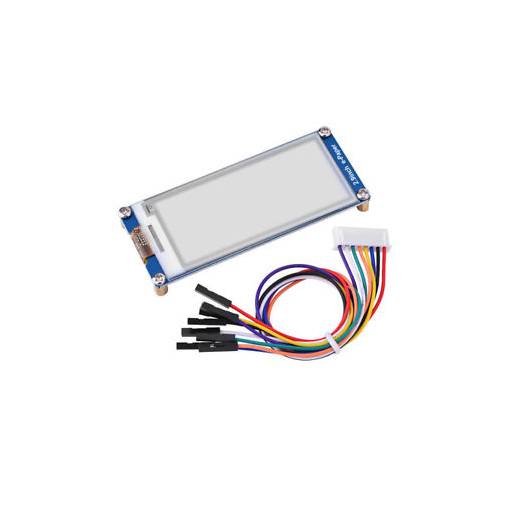
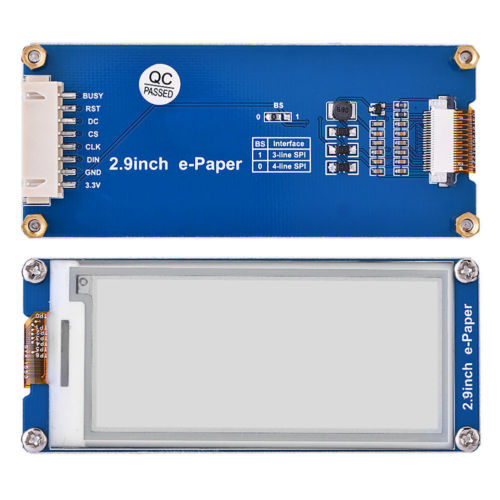
Zapojenie samotného displeja ku RP PICO
e-Paper | Pico | Description |
VCC | VSYS | Power input |
GND | GND | Ground |
DIN | GP11 | MOSI pin of SPI interface, data transmitted from Master to Slave. |
CLK | GP10 | SCK pin of SPI interface, clock input |
CS | GP9 | Chip select pin of SPI interface, Low Active |
DC | GP8 | Data/Command control pin (High: Data; Low: Command) |
RST | GP12 | Reset pin, low active |
BUSY | GP13 | Busy output pin |
KEY0 | GP2 | User key 0 |
KEY1 | GP3 | User key 1 |
RUN | RUN | Reset |
V prvom rade je potrebné importovať knižnicu. Pripojíme raspberry PICO a uložíme do neho nasledujúci kód pod názvom „pico_epaper.py“. Táto knižnica má jednu nevýhodu a to sú naše slovenské znaky s diakritikou.
# *****************************************************************************
# * | File : Pico_ePaper-2.9-C.py
# * | Author : Waveshare team
# * | Function : Electronic paper driver
# * | Info :
# *----------------
# * | This version: V1.0
# * | Date : 2021-03-16
# # | Info : python demo
# -----------------------------------------------------------------------------
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documnetation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS OR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
# THE SOFTWARE.
#
from machine import Pin, SPI
import framebuf
import utime
# Display resolution
EPD_WIDTH = 128 #128
EPD_HEIGHT = 296 #296
RST_PIN = 12
DC_PIN = 8
CS_PIN = 9
BUSY_PIN = 13
class EPD_2in9_C:
def __init__(self):
self.reset_pin = Pin(RST_PIN, Pin.OUT)
self.busy_pin = Pin(BUSY_PIN, Pin.IN, Pin.PULL_UP)
self.cs_pin = Pin(CS_PIN, Pin.OUT)
self.width = EPD_WIDTH
self.height = EPD_HEIGHT
self.spi = SPI(1)
self.spi.init(baudrate=4000_000)
self.dc_pin = Pin(DC_PIN, Pin.OUT)
self.buffer_black = bytearray(self.height * self.width // 8)
self.buffer_red = bytearray(self.height * self.width // 8)
self.imageblack = framebuf.FrameBuffer(self.buffer_black, self.width, self.height, framebuf.MONO_HLSB)
self.imagered = framebuf.FrameBuffer(self.buffer_red, self.width, self.height, framebuf.MONO_HLSB)
self.init()
def digital_write(self, pin, value):
pin.value(value)
def digital_read(self, pin):
return pin.value()
def delay_ms(self, delaytime):
utime.sleep(delaytime / 1000.0)
def spi_writebyte(self, data):
self.spi.write(bytearray(data))
def module_exit(self):
self.digital_write(self.reset_pin, 0)
# Hardware reset
def reset(self):
self.digital_write(self.reset_pin, 1)
self.delay_ms(50)
self.digital_write(self.reset_pin, 0)
self.delay_ms(2)
self.digital_write(self.reset_pin, 1)
self.delay_ms(50)
def send_command(self, command):
self.digital_write(self.dc_pin, 0)
self.digital_write(self.cs_pin, 0)
self.spi_writebyte([command])
self.digital_write(self.cs_pin, 1)
def send_data(self, data):
self.digital_write(self.dc_pin, 1)
self.digital_write(self.cs_pin, 0)
self.spi_writebyte([data])
self.digital_write(self.cs_pin, 1)
def send_data1(self, buf):
self.digital_write(self.dc_pin, 1)
self.digital_write(self.cs_pin, 0)
self.spi.write(bytearray(buf))
self.digital_write(self.cs_pin, 1)
def ReadBusy(self):
print('busy')
self.send_command(0x71)
while(self.digital_read(self.busy_pin) == 0):
self.send_command(0x71)
self.delay_ms(10)
print('busy release')
def TurnOnDisplay(self):
self.send_command(0x12)
self.ReadBusy()
def init(self):
print('init')
self.reset()
self.send_command(0x06) # boost
self.send_data (0x17)
self.send_data (0x17)
self.send_data (0x17)
self.send_command(0x04) # POWER_ON
self.ReadBusy()
self.send_command(0X00) # PANEL_SETTING
self.send_data(0x8F)
self.send_command(0X50) # VCOM_AND_DATA_INTERVAL_SETTING
self.send_data(0x77)
self.send_command(0x61) # TCON_RESOLUTION
self.send_data (0x80)
self.send_data (0x01)
self.send_data (0x28)
return 0
def display(self):
self.send_command(0x10)
self.send_data1(self.buffer_black)
self.send_command(0x13)
self.send_data1(self.buffer_red)
self.TurnOnDisplay()
def Clear(self, colorblack, colorred):
self.send_command(0x10)
self.send_data1([colorred] * self.height * int(self.width / 8))
self.send_command(0x13)
self.send_data1([colorred] * self.height * int(self.width / 8))
self.TurnOnDisplay()
def sleep(self):
self.send_command(0X02) # power off
self.ReadBusy()
self.send_command(0X07) # deep sleep
self.send_data(0xA5)
self.delay_ms(2000)
self.module_exit()
if __name__=='__main__':
epd = EPD_2in9_C()
epd.Clear(0xff, 0xff)
epd.imageblack.fill(0xff)
epd.imagered.fill(0xff)
epd.imageblack.text("Waveshare", 0, 10, 0x00)
epd.imagered.text("ePaper-2.9-C", 0, 25, 0x00)
epd.imageblack.text("RPi Pico", 0, 40, 0x00)
epd.imagered.text("Hello World", 0, 55, 0x00)
epd.display()
epd.delay_ms(2000)
epd.imagered.vline(10, 90, 40, 0x00)
epd.imagered.vline(90, 90, 40, 0x00)
epd.imageblack.hline(10, 90, 80, 0x00)
epd.imageblack.hline(10, 130, 80, 0x00)
epd.imagered.line(10, 90, 90, 130, 0x00)
epd.imageblack.line(90, 90, 10, 130, 0x00)
epd.display()
epd.delay_ms(2000)
epd.imageblack.rect(10, 150, 40, 40, 0x00)
epd.imagered.fill_rect(60, 150, 40, 40, 0x00)
epd.display()
epd.delay_ms(2000)
epd.Clear(0xff, 0xff)
epd.delay_ms(2000)
print("sleep")
epd.sleep()
Aj táto knižnica je priamo spustiteľná, všimnite si riadok 165. Všetko od tohto riadku sa spustí, pokiaľ je knižnica volaná priamo a neodkazuje sa len na ňu.
Ak budeme chcieť vytvoriť spustiteľné demo, môžeme to urobiť nasledovne (uložíme ho ako „main.py“):
# naimportujeme knižnicu
from pico_epaper import EPD_2in9_C
# www.tkinter.eu
print("www.tkinter.eu")
while True:
# vytvoríme si inštanciu, ktorou budeme mať prístup ku všetkým metódam
epd = EPD_2in9_C()
epd.Clear(0xff, 0xff)
epd.imageblack.fill(0xff)
epd.imagered.fill(0xff)
epd.imageblack.text("Waveshare", 0, 10, 0x00)
epd.imagered.text("ePaper-2.9-C", 0, 25, 0x00)
epd.imageblack.text("RPi Pico", 0, 40, 0x00)
epd.imagered.text("Hello World", 0, 55, 0x00)
epd.display()
epd.delay_ms(2000)
epd.imagered.vline(10, 90, 40, 0x00)
epd.imagered.vline(90, 90, 40, 0x00)
epd.imageblack.hline(10, 90, 80, 0x00)
epd.imageblack.hline(10, 130, 80, 0x00)
epd.imagered.line(10, 90, 90, 130, 0x00)
epd.imageblack.line(90, 90, 10, 130, 0x00)
epd.display()
epd.delay_ms(2000)
epd.imageblack.rect(10, 150, 40, 40, 0x00)
epd.imagered.fill_rect(60, 150, 40, 40, 0x00)
epd.display()
epd.delay_ms(2000)
epd.Clear(0xff, 0xff)
epd.delay_ms(2000)
print("sleep")
epd.sleep()
Samotné vykreslenie na displeji trvá cca 20 – 30 sekúnd, kontrast a zobrazenie je výborné, spotreba skoro žiadna.